Connect to iJewel Drive
The setupIjewelDriveViewer
function is a convenient way to set up the iJewel3D Viewer by connecting it to your iJewel drive. This allows you to load a 3D model directly from a specified path inside your ijewel drive and render it within your web application. Here's how to use it.
Getting Started
To get started quickly, go to your iJewel drive and navigate to the viewer
folder. Inside this folder, you will find an index.html
file that is pre-configured to work with your drive. This file serves as an example of how to embed the iJewel3D Viewer and connect it to your drive. You can open this file in your browser to see the viewer in action and refer to its setup when integrating the viewer into your own web application.
TIP
You can download the source code of the above demo from the link bellow
view-source:https://releases.ijewel3d.com/libs/mini-viewer/index.html?path=L3Rlc3QvcmluZy5nbGI%3D
Usage
To initialize the viewer, call the setupIjewelDriveViewer
function with the necessary configuration. Here's an example:
ijewelViewer.setupIjewelDriveViewer({
basePath: "https://assets.example.ijewel3d.com",
ijewelViewerOptions: {
showLogo: true,
logoScale: 1,
// Additional options...
},
});
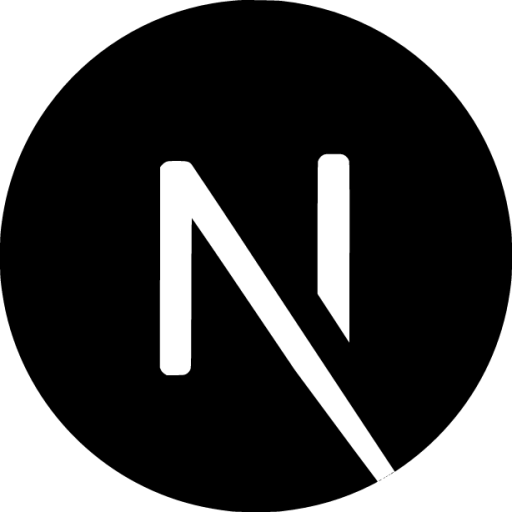
NextJS Example
useEffect(() => {
const script = document.createElement("script");
script.src = "https://releases.ijewel3d.com/libs/mini-viewer/0.1.1/bundle.iife.js";
script.async = true;
script.onload = () => {
//set it to true during development to bypass cors.
const useProxy = false;
const baseName = 'yourBaseName';
const basePath = "https://assets." + baseName + ".ijewel3d.com";
const proxyUrl = useProxy ? "https://cors-proxy.r2cache.com/" : "";
// @ts-ignore
ijewelViewer.setupIjewelDriveViewer({
basePath: proxyUrl + basePath,
ijewelViewerOptions: {
showCard: true,
showLogo: true,
},
}, document.getElementById("wrapper"));
};
document.body.appendChild(script);
}, []);
Parameters
Parameter | Type | Description | Default |
---|---|---|---|
basePath | string | The base URL path where your assets are stored. | N/A |
model (Optional) | string | The relative path to the 3D model file inside your iJewel Drive. For instance, if your model is located in a folder named 3dFolder and the file is called model.glb , you would specify the path as /3dFolder/model.glb . This path is relative to the basePath provided in the configuration. It can be left undefined and the model will be taken from the query parameter path inside your iJewel Drive. | N/A |
target | HTMLElement | (Optional) The HTML element where the viewer will be rendered. | null |
ijewelViewerOptions | object | An object with viewer options such as showLogo, logoScale, etc. | N/A |
useMetaConfig | boolean | Whether to use the saved meta configuration file for the 3d model. | true |
useFolderConfig | boolean | Whether to use the parent folder configuration file. | false |
useDefaultConfig | boolean | Whether to use the main default configuration file. | true |
Setting the Model Path via URL Query Parameter
You can use the query parameter path
to tell the viewer which model to load. The path to the model should be in Base64 format and relative to the root folder in your iJewel Drive. For example:
www.yourwebsite.com/?path=L3Rlc3QvcmluZy5nbGI
In this example, the Base64-encoded string L3Rlc3QvcmluZy5nbGI
decodes to /test/ring.glb
, which is the path to the 3D model, once you initilize iJewel Viewer, it will refer to this path to know which model to load.
Details
Alternatively, you can directly set the model path using the model property in the setupIjewelDriveViewer
method. This provides a flexible way to specify the model path either through the URL or within your JavaScript code.
INFO
Once you call setupIjewelDriveViewer
with the correct model path, it will take care of loading the specified 3D model along with all its associated settings. This includes any predefined configurations, materials, and environment settings that have been saved with the model. This seamless integration ensures consistency and ease of access to your models. You can edit your models directly in the drive, save your changes, and see those updates reflected instantly on your website.
Cross-Origin Resource Sharing (CORS)
CORS is setup on the iJewel Drive to make sure only you can host the models on your domain.
When connecting to your iJewel Drive and loading models, you may encounter Cross-Origin Resource Sharing (CORS) errors in the console. These issues arise because your domain hadn't been whitelisted, please reach to us so we can configure our servers to allow CORS for your domain.
TIP
For development and staging, a CORS-proxy can be used. Check the pre-configured sample in your iJewel Drive instance on how to use it. Note that this would disable the caching of the files on the CDN and adds a rate limit to the number of requests along with some other checks. Therefore, make sure to disable the flag when using in production to make the viewer running smoothly.